Example - 3 day full body¶
This notebook shows features of streprogen, the Python strength program generator.
Contributions to the code are welcome. :)
[1]:
!pip install streprogen matplotlib --quiet
[2]:
import functools
import matplotlib.pyplot as plt
[3]:
from streprogen import Program
from streprogen.utils import round_to_nearest
from streprogen.modeling import (reps_to_intensity_tight,
progression_diffeq,
progression_sawtooth)
[4]:
duration = 16
percent_inc_per_week = 1
period = 4
Create a custom sawtooth progress function with deloads¶
[5]:
def progression_sawtooth_clipped(week, start_weight,
final_weight, start_week, final_week, **kwargs):
"""Clipped progress function with deloading."""
# Standard sawtooth progression function from streprogen
ans = progression_sawtooth(week,
start_weight,
final_weight,
start_week,
final_week, **kwargs)
# Every `period` weeks, add a deloading week
if (week - 1) % period == 0:
ans = ans * 0.97 # Deload factor
# Clip the results and and return
return max(start_weight * 0.99, min(ans, final_weight * 1.01))
[6]:
# Parameters for the plot
weeks = list(range(1, duration+1))
start_weight = 100
final_weight = round(100 * (1 + duration * (percent_inc_per_week / 100)))
# Evaluate the function
params = {"period":period, "scale":0.022, "offset":0, "k":1.1}
y = [progression_sawtooth_clipped(
w,
start_weight=start_weight,
final_weight=final_weight,
start_week=1,
final_week=duration,
**params
) for w in weeks]
# Plot the general strength progression function
plt.title("Strength progression")
plt.plot(weeks, y, '-o')
plt.grid(True)
plt.show()
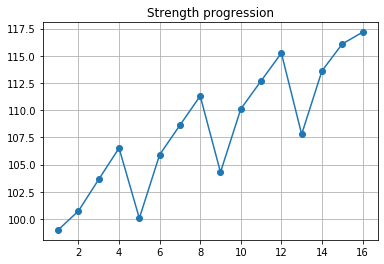
[7]:
progress = functools.partial(progression_sawtooth_clipped, **params)
Set up scaling functions for intensity and repetitions¶
[8]:
params = {"start_weight":1.0,
"final_weight":1.1,
"start_week":1,
"final_week":duration,
"period":period,
"scale":0.03}
intensity_scalers = [progression_sawtooth_clipped(w, **params) for w in weeks]
intensity_scaler_func = functools.partial(progression_sawtooth_clipped, **params)
params = {"start_weight":1 - 0.25,
"final_weight":1 + 0.25,
"start_week":1,
"final_week":duration,
"period":period,
"scale":0.2}
def inv_sawtooth(*args, **kwargs):
return 2 - progression_sawtooth(*args, **kwargs)
rep_scalers = [inv_sawtooth(w, **params) for w in weeks]
rep_scaler_func = functools.partial(inv_sawtooth, **params)
# Create a plot
plt.plot(weeks, intensity_scalers, '-o', label="intensity_scalers")
plt.plot(weeks, rep_scalers, '-o', label="rep_scalers")
plt.legend()
plt.grid(True)
plt.show()
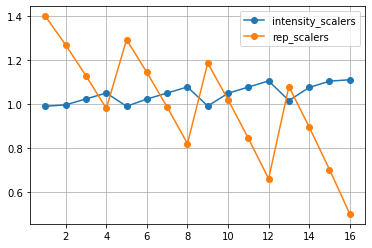
Program setup¶
Below is the code creating the program.
[9]:
program = Program(name=f'3days{duration}weeks',
duration=duration,
percent_inc_per_week=percent_inc_per_week,
reps_per_exercise=22,
rep_scaler_func=rep_scaler_func,
intensity=reps_to_intensity_tight(6),
intensity_scaler_func=intensity_scaler_func,
progression_func=progress,
# Using the 'tight' function instead of the standard one
reps_to_intensity_func=reps_to_intensity_tight,
units='',
round_to=2.5,
verbose=True)
def lunges(w):
weight = 24 + ((w - 1) // 2) * 2
sets = 3 if (w % 2) else 4
weight = round_to_nearest(weight, 2)
return f"{sets} x 6 @ {weight} kg"
def military(w):
weight = 55 + ((w - 1) // 2) * 2.5
sets = 3 if (w % 2) else 4
weight = round_to_nearest(weight, 2.5)
return f"{sets} x 6 @ {weight} kg"
def deadlifts(w):
weight = progression_diffeq(
week=w,
start_weight=80,
final_weight=150,
start_week=1,
final_week=duration,
k=2,
)
weight = round_to_nearest(weight, 2.5)
return f"3 x 3 @ {weight} kg"
with program.Day("Day 1"):
program.DynamicExercise("Bench press", start_weight=140, min_reps=2, max_reps=7)
program.StaticExercise('Lunges', lunges)
program.StaticExercise('Deadlifts', deadlifts)
with program.Day("Day 2"):
program.DynamicExercise(name="Narrow-grip bench", start_weight=130, min_reps=2, max_reps=7)
# My bodyweight is 85 kilograms, so I use (85 + 60) as the start weight for
# chin ups. This means that my 1RM is 60kg in the belt.
program.DynamicExercise(name="Chins 85kg+ (heavy)", start_weight=(85 + 60),
min_reps=2, max_reps=7, round_to=5)
program.StaticExercise('Dumbbell rows', "4 x 10 @ 40kg")
with program.Day("Day 3"):
program.DynamicExercise(name="Chins 85kg+ (light)", start_weight=(85 + 45),
min_reps=2, max_reps=7, round_to=5)
program.DynamicExercise(name="Front squats", start_weight=120, final_weight=150,
min_reps=3, max_reps=7, intensity=82)
program.StaticExercise("Military press", military)
Render the program¶
[10]:
# Do the computations and render a program. Might take a few seconds.
program.render()
Rendered program in 0.09 seconds.
/home/tommy/Desktop/github/streprogen/streprogen/program.py:262: UserWarning:
WARNING: Average intensity is > 90.
warnings.warn("\nWARNING: Average intensity is > 90.")
/home/tommy/Desktop/github/streprogen/streprogen/program.py:273: UserWarning:
WARNING: Number of repetitions < 15.
warnings.warn("\nWARNING: Number of repetitions < 15.")
[11]:
print(program)
----------------------------------------------------------------
Program: 3days16weeks
Program parameters
duration: 16
reps_per_exercise: 22
intensity: 82.5
units:
----------------------------------------------------------------
Exercise information
Day 1
Bench press 140 -> 162.4
reps: [2, 7] weekly inc.: 1.0%
Lunges 3 x 6 @ 24 kg
Deadlifts 3 x 3 @ 80 kg
Day 2
Narrow-grip bench 130 -> 150.8
reps: [2, 7] weekly inc.: 1.0%
Chins 85kg+ (heavy) 145 -> 168.2
reps: [2, 7] weekly inc.: 1.0%
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 130 -> 150.8
reps: [2, 7] weekly inc.: 1.0%
Front squats 120 -> 150
reps: [3, 7] weekly inc.: 1.6%
Military press 3 x 6 @ 55 kg
----------------------------------------------------------------
Program
Week 1
Day 1
Bench press 7 x 110 7 x 110 6 x 115 6 x 115 5 x 117.5
Lunges 3 x 6 @ 24 kg
Deadlifts 3 x 3 @ 80 kg
Day 2
Narrow-grip bench 7 x 102.5 7 x 102.5 6 x 105 6 x 105 5 x 110
Chins 85kg+ (heavy) 7 x 115 7 x 115 6 x 120 6 x 120 5 x 120
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 7 x 105 7 x 105 6 x 105 6 x 105 5 x 110
Front squats 7 x 95 7 x 95 6 x 97.5 6 x 97.5 5 x 102.5
Military press 3 x 6 @ 55 kg
Week 2
Day 1
Bench press 7 x 112.5 6 x 117.5 5 x 120 5 x 120 5 x 120
Lunges 4 x 6 @ 24 kg
Deadlifts 3 x 3 @ 90 kg
Day 2
Narrow-grip bench 7 x 105 6 x 107.5 5 x 112.5 5 x 112.5 5 x 112.5
Chins 85kg+ (heavy) 7 x 115 6 x 120 5 x 125 5 x 125 5 x 125
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 7 x 105 6 x 110 5 x 110 5 x 110 5 x 110
Front squats 7 x 97.5 6 x 100 6 x 100 5 x 105 5 x 105
Military press 4 x 6 @ 55 kg
Week 3
Day 1
Bench press 6 x 120 6 x 120 5 x 125 4 x 127.5 4 x 127.5
Lunges 3 x 6 @ 26 kg
Deadlifts 3 x 3 @ 97.5 kg
Day 2
Narrow-grip bench 6 x 110 6 x 110 5 x 115 4 x 120 4 x 120
Chins 85kg+ (heavy) 6 x 125 6 x 125 5 x 130 4 x 135 4 x 135
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 6 x 110 6 x 110 5 x 115 4 x 120 4 x 120
Front squats 6 x 105 6 x 105 5 x 107.5 4 x 112.5 4 x 112.5
Military press 3 x 6 @ 57.5 kg
Week 4
Day 1
Bench press 5 x 127.5 5 x 127.5 5 x 127.5 4 x 132.5 3 x 135
Lunges 4 x 6 @ 26 kg
Deadlifts 3 x 3 @ 105 kg
Day 2
Narrow-grip bench 5 x 117.5 5 x 117.5 5 x 117.5 4 x 122.5 3 x 127.5
Chins 85kg+ (heavy) 5 x 130 5 x 130 5 x 130 4 x 135 3 x 140
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 5 x 120 5 x 120 5 x 120 4 x 120 3 x 125
Front squats 5 x 112.5 5 x 112.5 5 x 112.5 4 x 115 3 x 120
Military press 4 x 6 @ 57.5 kg
Week 5
Day 1
Bench press 7 x 112.5 6 x 115 6 x 115 5 x 120 5 x 120
Lunges 3 x 6 @ 28 kg
Deadlifts 3 x 3 @ 112.5 kg
Day 2
Narrow-grip bench 7 x 105 6 x 107.5 6 x 107.5 5 x 110 5 x 110
Chins 85kg+ (heavy) 7 x 115 6 x 120 6 x 120 5 x 125 5 x 125
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 7 x 105 6 x 105 6 x 105 5 x 110 5 x 110
Front squats 7 x 97.5 7 x 97.5 7 x 97.5 6 x 102.5
Military press 3 x 6 @ 60 kg
Week 6
Day 1
Bench press 6 x 122.5 6 x 122.5 5 x 127.5 4 x 130 4 x 130
Lunges 4 x 6 @ 28 kg
Deadlifts 3 x 3 @ 117.5 kg
Day 2
Narrow-grip bench 6 x 112.5 6 x 112.5 5 x 117.5 4 x 122.5 4 x 122.5
Chins 85kg+ (heavy) 6 x 125 6 x 125 5 x 130 4 x 135 4 x 135
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 6 x 115 6 x 115 5 x 115 4 x 120 4 x 120
Front squats 6 x 107.5 6 x 107.5 5 x 112.5 4 x 115 4 x 115
Military press 4 x 6 @ 60 kg
Week 7
Day 1
Bench press 5 x 130 5 x 130 5 x 130 4 x 135 3 x 137.5
Lunges 3 x 6 @ 30 kg
Deadlifts 3 x 3 @ 122.5 kg
Day 2
Narrow-grip bench 5 x 120 5 x 120 5 x 120 4 x 125 3 x 130
Chins 85kg+ (heavy) 5 x 135 5 x 135 5 x 135 4 x 140 3 x 145
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 5 x 120 5 x 120 5 x 120 4 x 125 3 x 130
Front squats 5 x 115 5 x 115 5 x 115 4 x 120 3 x 122.5
Military press 3 x 6 @ 62.5 kg
Week 8
Day 1
Bench press 5 x 132.5 4 x 137.5 3 x 142.5 3 x 142.5 3 x 142.5
Lunges 4 x 6 @ 30 kg
Deadlifts 3 x 3 @ 127.5 kg
Day 2
Narrow-grip bench 5 x 122.5 4 x 127.5 3 x 132.5 3 x 132.5 3 x 132.5
Chins 85kg+ (heavy) 5 x 140 4 x 140 3 x 145 3 x 145 3 x 145
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 5 x 125 4 x 130 3 x 130 3 x 130 3 x 130
Front squats 5 x 120 4 x 122.5 3 x 127.5 3 x 127.5 3 x 127.5
Military press 4 x 6 @ 62.5 kg
Week 9
Day 1
Bench press 7 x 117.5 7 x 117.5 6 x 120 6 x 120
Lunges 3 x 6 @ 32 kg
Deadlifts 3 x 3 @ 130 kg
Day 2
Narrow-grip bench 7 x 107.5 7 x 107.5 6 x 112.5 6 x 112.5
Chins 85kg+ (heavy) 7 x 120 7 x 120 6 x 125 6 x 125
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 7 x 110 7 x 110 6 x 110 6 x 110
Front squats 7 x 105 7 x 105 6 x 107.5 6 x 107.5
Military press 3 x 6 @ 65 kg
Week 10
Day 1
Bench press 5 x 132.5 5 x 132.5 5 x 132.5 4 x 135 3 x 140
Lunges 4 x 6 @ 32 kg
Deadlifts 3 x 3 @ 135 kg
Day 2
Narrow-grip bench 5 x 122.5 5 x 122.5 5 x 122.5 4 x 127.5 3 x 130
Chins 85kg+ (heavy) 5 x 135 5 x 135 5 x 135 4 x 140 3 x 145
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 5 x 120 5 x 120 5 x 120 4 x 125 3 x 130
Front squats 5 x 120 5 x 120 5 x 120 4 x 122.5 3 x 127.5
Military press 4 x 6 @ 65 kg
Week 11
Day 1
Bench press 4 x 140 4 x 140 4 x 140 4 x 140 3 x 145
Lunges 3 x 6 @ 34 kg
Deadlifts 3 x 3 @ 137.5 kg
Day 2
Narrow-grip bench 4 x 130 4 x 130 4 x 130 4 x 130 3 x 132.5
Chins 85kg+ (heavy) 4 x 145 4 x 145 4 x 145 4 x 145 3 x 150
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 4 x 130 4 x 130 4 x 130 4 x 130 3 x 135
Front squats 5 x 122.5 4 x 127.5 4 x 127.5 3 x 130 3 x 130
Military press 3 x 6 @ 67.5 kg
Week 12
Day 1
Bench press 3 x 147.5 3 x 147.5 3 x 147.5 3 x 147.5 3 x 147.5
Lunges 4 x 6 @ 34 kg
Deadlifts 3 x 3 @ 140 kg
Day 2
Narrow-grip bench 3 x 137.5 3 x 137.5 3 x 137.5 3 x 137.5 3 x 137.5
Chins 85kg+ (heavy) 3 x 150 3 x 150 3 x 150 3 x 150 3 x 150
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 3 x 135 3 x 135 3 x 135 3 x 135 3 x 135
Front squats 3 x 135 3 x 135 3 x 135 3 x 135 3 x 135
Military press 4 x 6 @ 67.5 kg
Week 13
Day 1
Bench press 6 x 125 6 x 125 6 x 125 5 x 127.5
Lunges 3 x 6 @ 36 kg
Deadlifts 3 x 3 @ 142.5 kg
Day 2
Narrow-grip bench 6 x 115 6 x 115 6 x 115 5 x 120
Chins 85kg+ (heavy) 6 x 130 6 x 130 6 x 130 5 x 135
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 6 x 115 6 x 115 6 x 115 5 x 120
Front squats 6 x 115 6 x 115 6 x 115 6 x 115
Military press 3 x 6 @ 70 kg
Week 14
Day 1
Bench press 4 x 140 4 x 140 4 x 140 4 x 140 4 x 140
Lunges 4 x 6 @ 36 kg
Deadlifts 3 x 3 @ 145 kg
Day 2
Narrow-grip bench 4 x 130 4 x 130 4 x 130 4 x 130 4 x 130
Chins 85kg+ (heavy) 4 x 145 4 x 145 4 x 145 4 x 145 4 x 145
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 4 x 130 4 x 130 4 x 130 4 x 130 4 x 130
Front squats 4 x 130 4 x 130 4 x 130 4 x 130 4 x 130
Military press 4 x 6 @ 70 kg
Week 15
Day 1
Bench press 3 x 147.5 3 x 147.5 3 x 147.5 3 x 147.5 3 x 147.5
Lunges 3 x 6 @ 38 kg
Deadlifts 3 x 3 @ 147.5 kg
Day 2
Narrow-grip bench 3 x 137.5 3 x 137.5 3 x 137.5 3 x 137.5 3 x 137.5
Chins 85kg+ (heavy) 3 x 155 3 x 155 3 x 155 3 x 155 3 x 155
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 3 x 140 3 x 140 3 x 140 3 x 140 3 x 140
Front squats 3 x 137.5 3 x 137.5 3 x 137.5 3 x 137.5 3 x 137.5
Military press 3 x 6 @ 72.5 kg
Week 16
Day 1
Bench press 3 x 150 3 x 150 3 x 150 2 x 155
Lunges 4 x 6 @ 38 kg
Deadlifts 3 x 3 @ 150 kg
Day 2
Narrow-grip bench 3 x 140 3 x 140 3 x 140 2 x 142.5
Chins 85kg+ (heavy) 3 x 155 3 x 155 3 x 155 2 x 160
Dumbbell rows 4 x 10 @ 40kg
Day 3
Chins 85kg+ (light) 3 x 140 3 x 140 3 x 140 2 x 145
Front squats 3 x 137.5 3 x 137.5 3 x 137.5 3 x 137.5
Military press 4 x 6 @ 72.5 kg
----------------------------------------------------------------
Export the program as .html
or .tex
, then to .pdf
¶
A .html
file can be printed directly from your browser, or printed to a .pdf
from your browser.
[12]:
# Save the program as a HTML file
with open(f'{program.name}.html', 'w', encoding='utf-8') as file:
# Control table width (number of sets) by passing the 'table_width' argument
file.write(program.to_html(table_width=8))
[13]:
# Save the program as a TEX file
with open(f'{program.name}.tex', 'w', encoding='utf-8') as file:
file.write(program.to_tex(table_width=8))
Use a .tex
to generate .pdf
if you have LaTeX installed, or use:
- latexbase.com from your browser.
[14]:
# If you have LaTeX installed on your system, you can render a program to .tex
# Alternatively, you can paste the LaTeX into: https://latexbase.com/
print(program.to_tex(table_width=8))
% -----------------------------------------------
% Package imports
% -----------------------------------------------
\documentclass[12pt, a4paper]{article}% 'twoside' for printing
\usepackage[utf8]{inputenc}% Allow input to be UTF-8
\usepackage[margin=2cm]{geometry}% May be used to set margins
% -----------------------------------------------
% Document start
% -----------------------------------------------
\begin{document}
\large
\section*{Program: 3days16weeks}
This program was made using \verb|streprogen|,
the Python strength program generator.
The latest version can be found at \\
\verb|https://pypi.python.org/pypi/streprogen/|.
\section*{Program parameters}
\begin{tabular}{l|l}
\textbf{Parameter} & \textbf{Value} \\ \hline
\verb|duration| & 16 \\
\verb|reps_per_exercise| & 22 \\
\verb|intensity| & 82.5 \\
\verb|units| &
\end{tabular}
\section*{Exercise information}
\begin{tabular}{llllll}
\textbf{Exercise} & \textbf{Start} & \textbf{End} & \textbf{Reps min}
& \textbf{Reps max} & \textbf{Weekly increase} \\ \hline
\textbf{ Day 1 } & & & & & \\ \hline
\hspace{0.5em}Bench press &
140 &
162.4 &
2 & 7 &
1.0\%\\
\hspace{0.5em}Lunges & \multicolumn{ 5 }{l}{ 3 x 6 @ 24 kg } \\
\hspace{0.5em}Deadlifts & \multicolumn{ 5 }{l}{ 3 x 3 @ 80 kg } \\
\textbf{ Day 2 } & & & & & \\ \hline
\hspace{0.5em}Narrow-grip bench &
130 &
150.8 &
2 & 7 &
1.0\%\\
\hspace{0.5em}Chins 85kg+ (heavy) &
145 &
168.2 &
2 & 7 &
1.0\%\\
\hspace{0.5em}Dumbbell rows & \multicolumn{ 5 }{l}{ 4 x 10 @ 40kg } \\
\textbf{ Day 3 } & & & & & \\ \hline
\hspace{0.5em}Chins 85kg+ (light) &
130 &
150.8 &
2 & 7 &
1.0\%\\
\hspace{0.5em}Front squats &
120 &
150 &
3 & 7 &
1.6\%\\
\hspace{0.5em}Military press & \multicolumn{ 5 }{l}{ 3 x 6 @ 55 kg } \\
\end{tabular}
\clearpage
\section*{Program}
\subsection*{\hspace{0.25em} Week 1 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 7 x 110
& 7 x 110
& 6 x 115
& 6 x 115
& 5 x 117.5
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 24 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 80 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 7 x 102.5
& 7 x 102.5
& 6 x 105
& 6 x 105
& 5 x 110
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 7 x 115
& 7 x 115
& 6 x 120
& 6 x 120
& 5 x 120
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 7 x 105
& 7 x 105
& 6 x 105
& 6 x 105
& 5 x 110
&
&
\\
\hspace{0.75em} Front squats
& 7 x 95
& 7 x 95
& 6 x 97.5
& 6 x 97.5
& 5 x 102.5
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 55 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 2 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 7 x 112.5
& 6 x 117.5
& 5 x 120
& 5 x 120
& 5 x 120
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 24 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 90 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 7 x 105
& 6 x 107.5
& 5 x 112.5
& 5 x 112.5
& 5 x 112.5
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 7 x 115
& 6 x 120
& 5 x 125
& 5 x 125
& 5 x 125
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 7 x 105
& 6 x 110
& 5 x 110
& 5 x 110
& 5 x 110
&
&
\\
\hspace{0.75em} Front squats
& 7 x 97.5
& 6 x 100
& 6 x 100
& 5 x 105
& 5 x 105
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 55 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 3 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 6 x 120
& 6 x 120
& 5 x 125
& 4 x 127.5
& 4 x 127.5
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 26 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 97.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 6 x 110
& 6 x 110
& 5 x 115
& 4 x 120
& 4 x 120
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 6 x 125
& 6 x 125
& 5 x 130
& 4 x 135
& 4 x 135
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 6 x 110
& 6 x 110
& 5 x 115
& 4 x 120
& 4 x 120
&
&
\\
\hspace{0.75em} Front squats
& 6 x 105
& 6 x 105
& 5 x 107.5
& 4 x 112.5
& 4 x 112.5
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 57.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 4 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 5 x 127.5
& 5 x 127.5
& 5 x 127.5
& 4 x 132.5
& 3 x 135
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 26 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 105 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 5 x 117.5
& 5 x 117.5
& 5 x 117.5
& 4 x 122.5
& 3 x 127.5
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 5 x 130
& 5 x 130
& 5 x 130
& 4 x 135
& 3 x 140
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 5 x 120
& 5 x 120
& 5 x 120
& 4 x 120
& 3 x 125
&
&
\\
\hspace{0.75em} Front squats
& 5 x 112.5
& 5 x 112.5
& 5 x 112.5
& 4 x 115
& 3 x 120
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 57.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 5 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 7 x 112.5
& 6 x 115
& 6 x 115
& 5 x 120
& 5 x 120
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 28 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 112.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 7 x 105
& 6 x 107.5
& 6 x 107.5
& 5 x 110
& 5 x 110
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 7 x 115
& 6 x 120
& 6 x 120
& 5 x 125
& 5 x 125
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 7 x 105
& 6 x 105
& 6 x 105
& 5 x 110
& 5 x 110
&
&
\\
\hspace{0.75em} Front squats
& 7 x 97.5
& 7 x 97.5
& 7 x 97.5
& 6 x 102.5
&
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 60 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 6 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 6 x 122.5
& 6 x 122.5
& 5 x 127.5
& 4 x 130
& 4 x 130
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 28 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 117.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 6 x 112.5
& 6 x 112.5
& 5 x 117.5
& 4 x 122.5
& 4 x 122.5
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 6 x 125
& 6 x 125
& 5 x 130
& 4 x 135
& 4 x 135
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 6 x 115
& 6 x 115
& 5 x 115
& 4 x 120
& 4 x 120
&
&
\\
\hspace{0.75em} Front squats
& 6 x 107.5
& 6 x 107.5
& 5 x 112.5
& 4 x 115
& 4 x 115
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 60 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 7 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 5 x 130
& 5 x 130
& 5 x 130
& 4 x 135
& 3 x 137.5
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 30 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 122.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 5 x 120
& 5 x 120
& 5 x 120
& 4 x 125
& 3 x 130
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 5 x 135
& 5 x 135
& 5 x 135
& 4 x 140
& 3 x 145
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 5 x 120
& 5 x 120
& 5 x 120
& 4 x 125
& 3 x 130
&
&
\\
\hspace{0.75em} Front squats
& 5 x 115
& 5 x 115
& 5 x 115
& 4 x 120
& 3 x 122.5
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 62.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 8 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 5 x 132.5
& 4 x 137.5
& 3 x 142.5
& 3 x 142.5
& 3 x 142.5
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 30 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 127.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 5 x 122.5
& 4 x 127.5
& 3 x 132.5
& 3 x 132.5
& 3 x 132.5
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 5 x 140
& 4 x 140
& 3 x 145
& 3 x 145
& 3 x 145
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 5 x 125
& 4 x 130
& 3 x 130
& 3 x 130
& 3 x 130
&
&
\\
\hspace{0.75em} Front squats
& 5 x 120
& 4 x 122.5
& 3 x 127.5
& 3 x 127.5
& 3 x 127.5
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 62.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 9 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 7 x 117.5
& 7 x 117.5
& 6 x 120
& 6 x 120
&
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 32 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 130 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 7 x 107.5
& 7 x 107.5
& 6 x 112.5
& 6 x 112.5
&
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 7 x 120
& 7 x 120
& 6 x 125
& 6 x 125
&
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 7 x 110
& 7 x 110
& 6 x 110
& 6 x 110
&
&
&
\\
\hspace{0.75em} Front squats
& 7 x 105
& 7 x 105
& 6 x 107.5
& 6 x 107.5
&
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 65 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 10 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 5 x 132.5
& 5 x 132.5
& 5 x 132.5
& 4 x 135
& 3 x 140
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 32 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 135 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 5 x 122.5
& 5 x 122.5
& 5 x 122.5
& 4 x 127.5
& 3 x 130
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 5 x 135
& 5 x 135
& 5 x 135
& 4 x 140
& 3 x 145
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 5 x 120
& 5 x 120
& 5 x 120
& 4 x 125
& 3 x 130
&
&
\\
\hspace{0.75em} Front squats
& 5 x 120
& 5 x 120
& 5 x 120
& 4 x 122.5
& 3 x 127.5
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 65 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 11 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 4 x 140
& 4 x 140
& 4 x 140
& 4 x 140
& 3 x 145
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 34 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 137.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
& 3 x 132.5
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 4 x 145
& 4 x 145
& 4 x 145
& 4 x 145
& 3 x 150
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
& 3 x 135
&
&
\\
\hspace{0.75em} Front squats
& 5 x 122.5
& 4 x 127.5
& 4 x 127.5
& 3 x 130
& 3 x 130
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 67.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 12 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 3 x 147.5
& 3 x 147.5
& 3 x 147.5
& 3 x 147.5
& 3 x 147.5
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 34 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 140 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 3 x 150
& 3 x 150
& 3 x 150
& 3 x 150
& 3 x 150
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 3 x 135
& 3 x 135
& 3 x 135
& 3 x 135
& 3 x 135
&
&
\\
\hspace{0.75em} Front squats
& 3 x 135
& 3 x 135
& 3 x 135
& 3 x 135
& 3 x 135
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 67.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 13 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 6 x 125
& 6 x 125
& 6 x 125
& 5 x 127.5
&
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 36 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 142.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 6 x 115
& 6 x 115
& 6 x 115
& 5 x 120
&
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 6 x 130
& 6 x 130
& 6 x 130
& 5 x 135
&
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 6 x 115
& 6 x 115
& 6 x 115
& 5 x 120
&
&
&
\\
\hspace{0.75em} Front squats
& 6 x 115
& 6 x 115
& 6 x 115
& 6 x 115
&
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 70 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 14 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 4 x 140
& 4 x 140
& 4 x 140
& 4 x 140
& 4 x 140
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 36 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 145 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 4 x 145
& 4 x 145
& 4 x 145
& 4 x 145
& 4 x 145
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
&
&
\\
\hspace{0.75em} Front squats
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
& 4 x 130
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 70 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 15 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 3 x 147.5
& 3 x 147.5
& 3 x 147.5
& 3 x 147.5
& 3 x 147.5
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 3 x 6 @ 38 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 147.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 3 x 155
& 3 x 155
& 3 x 155
& 3 x 155
& 3 x 155
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 3 x 140
& 3 x 140
& 3 x 140
& 3 x 140
& 3 x 140
&
&
\\
\hspace{0.75em} Front squats
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 3 x 6 @ 72.5 kg } \\
\end{tabular}
\subsection*{\hspace{0.25em} Week 16 }
\subsection*{\hspace{0.5em} Day 1 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Bench press
& 3 x 150
& 3 x 150
& 3 x 150
& 2 x 155
&
&
&
\\
\hspace{0.75em} Lunges & \multicolumn{ 7 }{l}{ 4 x 6 @ 38 kg } \\
\hspace{0.75em} Deadlifts & \multicolumn{ 7 }{l}{ 3 x 3 @ 150 kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 2 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Narrow-grip bench
& 3 x 140
& 3 x 140
& 3 x 140
& 2 x 142.5
&
&
&
\\
\hspace{0.75em} Chins 85kg+ (heavy)
& 3 x 155
& 3 x 155
& 3 x 155
& 2 x 160
&
&
&
\\
\hspace{0.75em} Dumbbell rows & \multicolumn{ 7 }{l}{ 4 x 10 @ 40kg } \\
\end{tabular}
\subsection*{\hspace{0.5em} Day 3 }
\begin{tabular}{l|lllllll}
\hspace{0.75em} \textbf{Exercise} & \multicolumn{ 7 }{l}{ \textbf{Sets / reps} } \\ \hline
\hspace{0.75em} Chins 85kg+ (light)
& 3 x 140
& 3 x 140
& 3 x 140
& 2 x 145
&
&
&
\\
\hspace{0.75em} Front squats
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
& 3 x 137.5
&
&
&
\\
\hspace{0.75em} Military press & \multicolumn{ 7 }{l}{ 4 x 6 @ 72.5 kg } \\
\end{tabular}
\end{document}